Take the Pain out of Naming Variables with 3 Simple Steps!
A cheat sheet for choosing good names and avoiding the bad ones
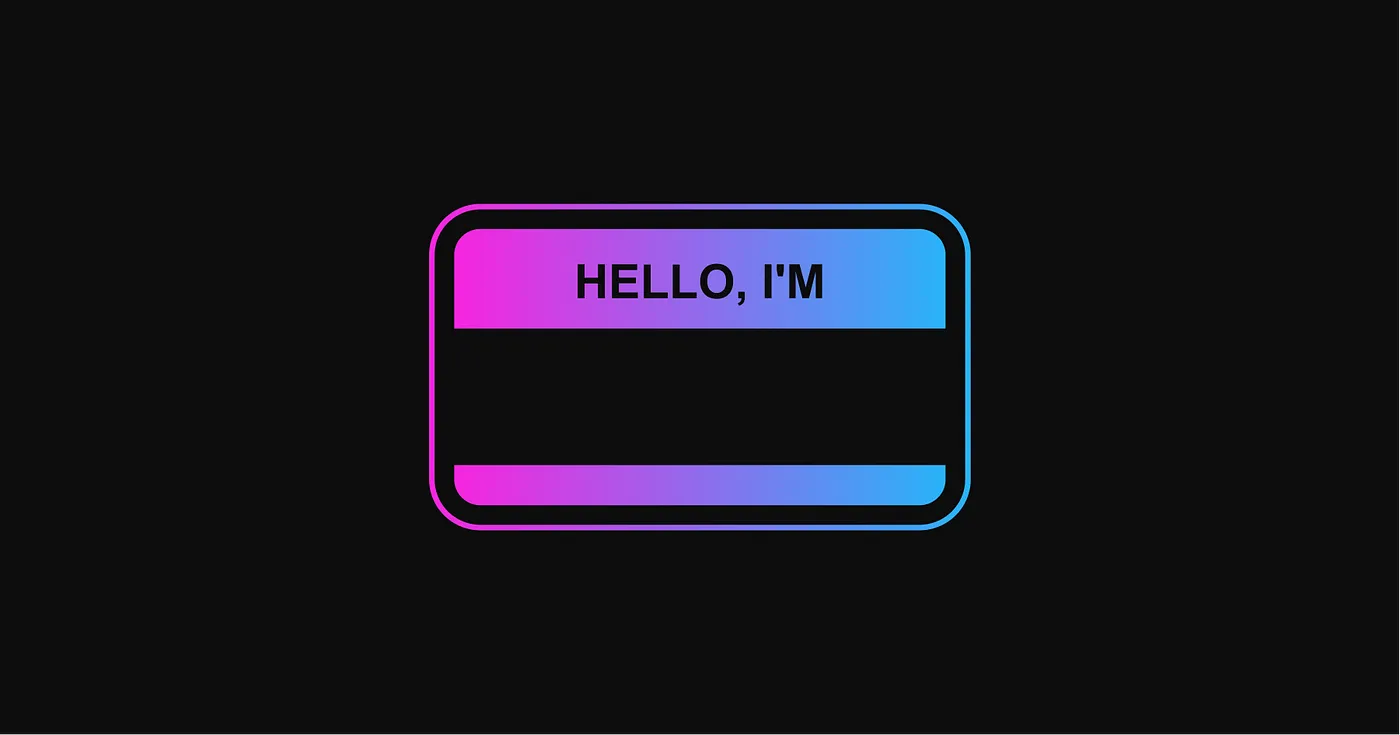
You know good and bad function names when you see them in someone else’s code, right? So then why is it so hard to come up with names for things in our code?? We waste so much time staring at that blinking cursor on the screen while we try to come up with the perfect name for something.
Perhaps the reason it is so hard to name things in our code is that we don’t actually understand what our code is going to do until after we have written it. I figured out a few tricks that cut the naming pain out of my process and I wanted to share them with you! This could change your life!
Summary / TL;DR
- Good names: tells what the thing does and follows the Single Responsibility Principle (SRP). Generally, less is more here.
- Bad name red flags: contains multiple conjunctions (i.e. and, or, but, for, etc); reads like a user story; does not describe what the thing does.
- The secret to naming things: don’t waste time. Call it something arbitrary (ThingOne, ServiceOne, userArray, etc) and start writing your code. Come back and fix the name after you actually know what the code is going to do.

- Meaningful. A good name tells what something does. It is an expression of the developer’s expectations and assumptions related to a specific piece of code.
- Self-explanatory. Good names typically do not require special explanation outside of business-specific terminology. Most good names don’t need comments to explain them.
- Follow the Single Responsibility Principle (SRP). Each class or method should be responsible for doing only one job, therefore their name should reflect that single responsibility. You usually end up with short names when you follow SRP, although that does NOT necessarily mean that a long name is a bad name.
let velocity: float;
const firstName: string;
public getUserPreferences(userId: string)
private removeDuplicates(myThings: Thing[])
public digestDonuts()
Good names also follow established patterns. Here are some examples:
- Javascript and Typescript function names should be camel-case (ex. myFunctionName())
- methods that invoke REST calls should use appropriate REST verbs (ex. putUserPreferences(), getShippingAddress(), postTakeoutMenuUpdate())
- No 3rd party names (usually). What happens to your getMapQuestDirections() method name if MapQuest isn’t around anymore? Better to call it getDirections() or getDrivingDirections()
Bad Names and How to Fix Them

My name is growing all the time, and I’ve lived a very long, long time; so my name is like a story. Real names tell you the story of the things they belong to in my language….
The Two Towers, Tolkien
🚩 RED FLAG: Given-When-Then or Act-When-If formatDoes the name sound like a user story description?
sendMarketingEmailsWhenOrderShipsIfOptedIn()
That’s a bad name! This function name has too many unnecessary details.
Let’s fix it!
Trim out the details and the conditional language: sendMarketingEmails(). Keep execution details inside the body of the function, not in the name.
🚩 RED FLAG: Multiple conjunctions (and, for, but, or, etc)
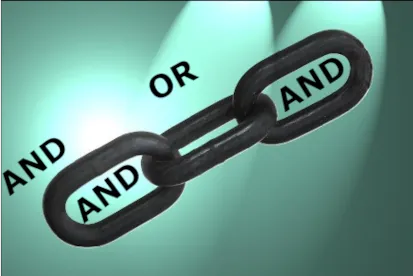
Does the name sound like a whole sentence?
checkUserCredentialsAndLogThemInIfAuthenticatedThenFetchPreferencesAndRouteToDashboardOrRouteToLoginIfNotAuthenticated()
That’s a bad name! Conjunctions are used to connect independent clauses in English. Multiple conjunctions in your method name means you are connecting multiple independent things, which means your method has multiple responsibilities.
Let’s fix it!
Split it into single responsibilities: authenticateUser(), getPreferences(), route().
🚩 RED FLAG: The name doesn’t describe the codeWhat if you run into something like this:
private deleteShippingAddress(userId: string, address: ShippingAddress): void {
const user: User = this.userService.getUser(userId);
user.addresses.forEach((a) => {
if(a === address) {
a.setInactive();
}
});
}
This code doesn’t actually delete an address. Maybe years ago when this code was first written it used to make an http call and delete an address, but right now it doesn’t. The name is inaccurate.
Let’s fix it!
Rename this function something like inactivateShippingAddress.
Naming Cheat Sheet For Everyone!
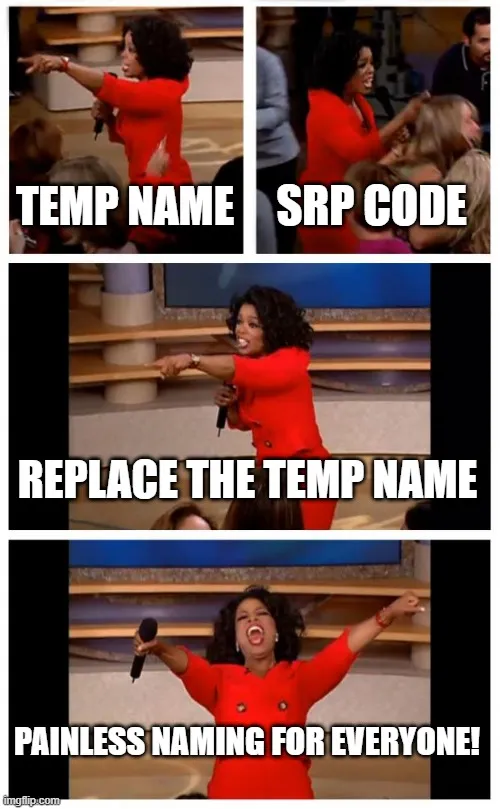
1. Use a temporary nameLook, if you don’t already know what you want to call this function or class or variable by the time your fingers hit the keyboard, don’t expect the name to magically come to you. Use a throw-away name and change it later.
let thingOne, elvis, lukeSkywalker, tempyVar, myFavoriteBandName;
public whatever() ...
public myTempMethod() ...
private changeThisNameLater() ...
2. Write your Single Responsibility codeThis is where you should be investing your time and brain power anyway!
3. ⚠️⚠️⚠️️️Replace the temporary name ⚠️⚠️⚠️After you are satisfied with the code you wrote, go back and change the throw-away name. If you wrote a function with only one job to do, you’ll know exactly what to name that function now!
That’s it! No pain needed. I used to lose SO MUCH TIME trying to figure out what to call my services and functions. Not anymore. I usually don’t know a perfect name when I start writing my code so I just throw whatever() or let tempyHama into my IDE and keep moving. I tell you, this has changed my life as a programmer. I hope it does the same for you.
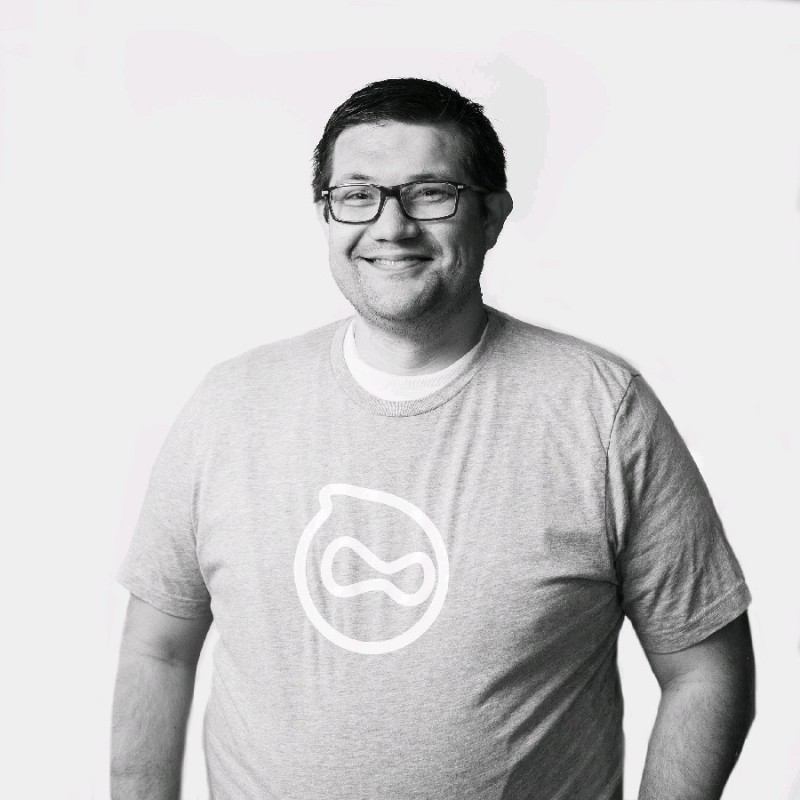